In this PHP tutorial, you shall learn about Two-dimensional Arrays, how to created 2D arrays, access elements of 2D arrays, and traverse the inner arrays of a 2D array, with example programs.
Multi-dimensional Arrays in PHP
Multi-dimensional Arrays are the arrays in which one or more elements of the array could be arrays.
The number of dimensions depends on the how deep the elements, of arrays, are arrays.
For example, if you take a One-dimensional array, the array contains elements but no arrays.
array(52, 41, 89, 63)
For Two-dimensional array, the array contains elements in which one or more elements of it are arrays. In total, the arrays are two-level deep.
array( array(7, 8, 9), array(4, 5, 6), array(1, 2, 3) )
Similarly, for Three-dimensional array, the outer array contains arrays as elements. These second level arrays, again contain arrays as elements. In total, three levels deep.
array( array( array(1, 0, 9), array(0, 5, 6), array(1, 0, 3) ), array( array(0, 4, 6), array(0, 0, 1), array(1, 2, 7) ), )
For N-dimensional array, the depth of arrays would be N.
Access N-dimensional Arrays
To access an N-dimensional array, we need N indexes to access an element.
The syntax to access an element in N-dimensional array would be as following.
$array[index_1][index_2][index_3]..[index_N]
One-Dimensional Arrays
An example for one-dimensional array in PHP is
array(52, 41, 89, 63)
As the number of dimensions is only one, we need only one index to access elements of it.
$array1D[index_1]
1. One-dimensional array with numbers
In the following example, we will create a one-dimensional array, and access its elements using index.
PHP Program
<?php $array1D = array(52, 41, 89, 63); echo $array1D[0]; echo "<br>"; echo $array1D[2]; ?>
Output
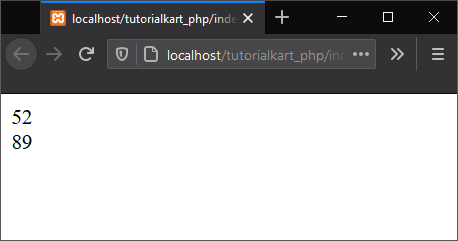
2. Iterate over Elements of 1-D Array using foreach
In the following example, we will create One-dimensional array, and print its elements using foreach statement.
PHP Program
<?php $array1D = array(52, 41, 89, 63); foreach ($array1D as $element) { echo $element; echo "<br>"; } ?>
Output
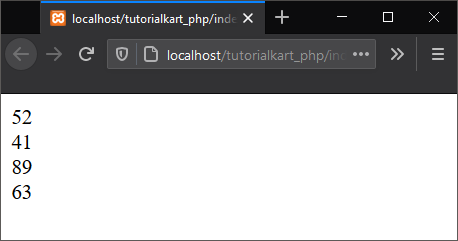
Two-Dimensional Arrays
An example for three-dimensional array in PHP is
array( array(7, 8, 9), array(4, 5, 6), array(1, 2, 3) );
As the number of dimensions is two, we need only one index to access elements of it.
$array2D[index_1][index_2]
1. Two-dimensional array with numbers
In the following example, we will create a two-dimensional array, and access the elements using index.
PHP Program
<?php $array2D = array( array(7, 8, 9), array(4, 5, 6), array(1, 2, 3) ); echo $array2D[0][1]; echo "<br>"; echo $array2D[2][1]; ?>
Output
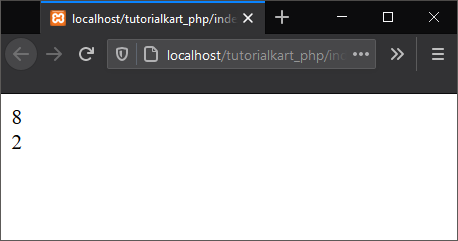
2. Iterate over elements of 2-D Array using foreach
In the following example, we will create a two-dimensional array, and print its elements using nested foreach.
PHP Program
<?php $array2D = array( array(7, 8, 9), array(4, 5, 6), array(1, 2, 3) ); foreach ($array2D as $array1D) { foreach ($array1D as $element) { echo $element; echo " "; } echo "<br>"; } ?>
Output
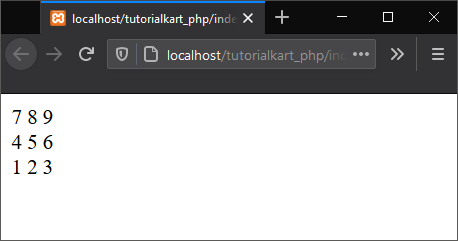
Three-Dimensional Arrays
An example for three-dimensional array is
array( array( array(1, 0, 9), array(0, 5, 6), array(1, 0, 3) ), array( array(0, 4, 6), array(0, 0, 1), array(1, 2, 7) ), )
As the number of dimensions is three, we need three index to access elements of it.
$array3D[index_1][index_2][index_3]
1. Three-dimensional array with numbers
In the following example, we will create a three-dimensional array, and access the elements using index.
PHP Program
<?php $array3D = array( array( array(1, 0, 9), array(0, 5, 6), array(1, 0, 3) ), array( array(0, 4, 6), array(0, 0, 1), array(1, 2, 7) ), ); echo $array3D[0][1][1]; echo "<br>"; echo $array3D[1][2][2]; ?>
Output
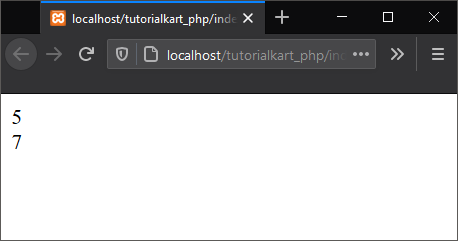
2. Iterate over elements of 3-D Array using foreach
In the following example, we will create a three-dimensional array, and print its elements using nested foreach.
PHP Program
<?php $array3D = array( array( array(1, 0, 9), array(0, 5, 6), array(1, 0, 3) ), array( array(0, 4, 6), array(0, 0, 1), array(1, 2, 7) ), ); foreach ($array3D as $array2D) { foreach ($array2D as $array1D) { foreach ($array1D as $element) { echo $element; echo " "; } echo "<br>"; } echo "<br>"; } ?>
Output
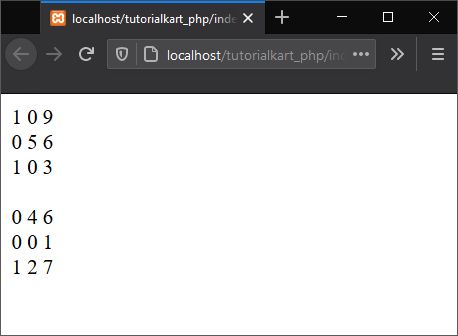
N-Dimensional Arrays
We can access or traverse through the elements of N-dimensional array in the same way as that of how we have done for 1D, 2D and 3D arrays.
Conclusion
In this PHP Tutorial, we learned how to create Multi-dimensional arrays, how to access the elements of Multi-dimensional arrays, and how to traverse through all the elements of Multi-dimensional arrays using nested foreach statement.