In this PHP tutorial, you shall learn how to convert a given string to an integer value using typecasting or intval() function, with example programs.
PHP – Convert String to Integer
To convert string to int in PHP, you can use Type Casting method or PHP built-in function intval().
In this tutorial, we will go through each of these methods and learn how to convert contents of a string to an integer value.
Convert String to Int using Type Casting
To convert string to integer using Type Casting, provide the literal (int)
along with parenthesis before the string literal. The expression returns integer value created from this string.
The syntax to type cast string to integer is
$int_value = (int) $string;
Example
In the following program, we take a string with integer content, and convert the string into integer using type casting.
PHP Program
<?php
$string = "41";
$int_value = (int) $string;
echo $int_value;
?>
Output
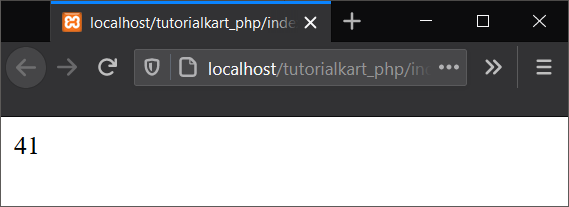
If the string contains a floating value, then the type casting trims out the decimal part.
In the following program, we take a string $x
which contains some decimal value. Then typecast this string to integer and observe the output.
PHP Program
<?php
$x = "3.14";
$n = (int) $x;
echo $n;
?>
Output
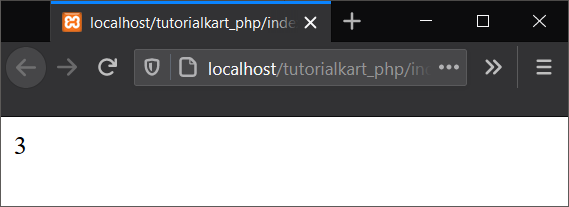
Convert String to Int using intval()
To convert string to integer using PHP intval() built-in function, pass the string as argument to the function. The function will return the integer value corresponding to the string content.
The syntax to use intval() to convert string to int is
$int_value = intval( $string );
Example
In the following example, we take a string value in $x
with integer content, and convert this string into an integer value using intval() function.
PHP Program
<?php
$x = "41";
$n = intval( $x );
echo $n;
?>
Output
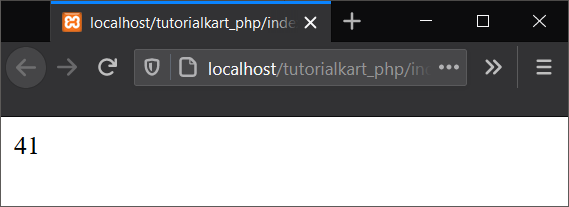
As observed in the type casting method, with intval() function value as well, you get the integer part even if the string contains some decimal point number.
PHP Program
<?php
$string = "3.14";
$int_value = intval( $string );
echo $int_value;
?>
Output
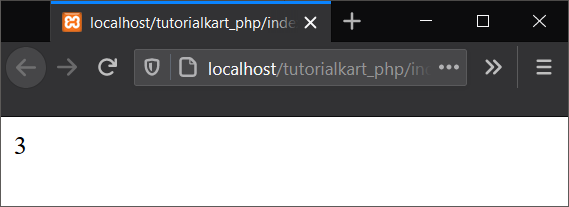
Conclusion
In this PHP Tutorial, we learned how to convert a string to int using type-casting or intval() function.