In this tutorial, you shall learn about functions in PHP, how to define a function, its syntax, with example programs.
Functions
In PHP, functions are blocks of code that can be executed multiple times throughout a script. They can be used to perform specific tasks or calculations, and they can accept arguments and return values.
Syntax of a function
The syntax of a function consists of the keyword function, followed by the function name. Then comma separated parameters enclosed in parenthesis, followed by a block of code enclosed in curly braces.
A simple example for a function is as shown in the following.
function add($a, $b) { $temp = $a + $b; return $a + $b; }
where
function
is the keyword that tells PHP that you’re defining a function. The keyword is followed by the name of the function.add
is the function name. This is the name you give your function. You should choose a name that reflects what the function does.($a, $b)
are the function parameters. These are variables that the function expects to receive as input. They are enclosed in parentheses after the function name. If there are multiple parameters, they are separated by commas.- The function body. This is the block of code that the function executes when it is called. It is enclosed in curly braces and can contain any number of statements. In this particular example, we have two statements: the first statement computes the sum of given two numbers, and the second is a return statement.
return $a + $b;
is the return statement. This is an optional statement that allows the function to return a value to the calling code. If you want your function to return a value, you include the return statement followed by the value you want to return.
Examples
1. Define a function that takes two parameters and return a value
In the following program, we write a function named add(), that expects two numbers as arguments, computes the sum of these two arguments, and returns the sum.
PHP Program
<?php function add($a, $b) { $temp = $a + $b; return $temp; } $result = add(4, 3); echo "The sum is : " . $result; ?>
Output
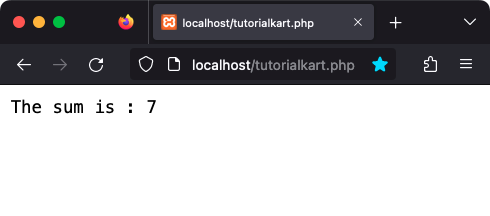
Conclusion
In this PHP Tutorial, we learned how to define functions, and how to write and call them in programs, with examples.