In this PHP tutorial, you shall learn how to check if a given string contains a specific word using strpos() function and if-else statement, with example programs.
PHP – Check if String Contains Specific Word
To check if string contains specific word, use strpos() function which finds the position of first occurrence of the specific word.
strpos($string, $word)
returns position of the word in string if the word is present in the string. If word is not present in the string, then strpos() returns false. So, we can write an if statement with the condition that the value returned by strpos() function is false. If the condition is true, the word is not present, else the word is present in the string.
Examples
1. Check if string contains the word “Apple”
In the following example, we will use strpos() function to check if string contains specific word.
PHP Program
<?php $str = "Apple is happy."; $word = "Apple"; if (strpos($str, $word) === false) { echo "Word is not present in string."; } else { echo "Word is present in string."; } ?>
Output
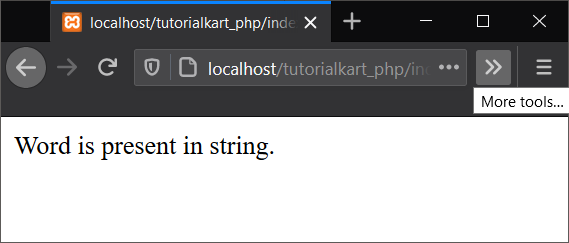
Conclusion
In this PHP Tutorial, we learned how to check if specific word is present in given string or not.