In this tutorial, you shall learn about PHP array_chunk() function which can split a given array into arrays of chunks with specific number of elements in each split, with syntax and examples.
PHP array_chunk() Function
PHP array_chunk() function splits the given array into arrays of chunks with specific number of elements.
You can mention the specific number of elements that has to go in each chunk.
Syntax of array_chunk()
The syntax of PHP array_chunk() function is
array_chunk ( array $array , int $size [, bool $preserve_keys = FALSE ] ) : array
where
Parameter | Description |
---|---|
array | The array which will be split into chunks. |
size | The number of elements in each chunk. |
preserve_keys | If TRUE, keys of the source array will be preserved.If FALSE, keys of the chunks will reindex. |
Return Value
The array_chunk() function returns an array of arrays, i.e., multi-dimensional array. Outer dimension is for the chunks, and the inner dimension is for the elements of the array.
Examples
1. Split given array into chunks of size 3
In this example, we will take an associative array with key-value pairs, and then split into chunks of size 3.
PHP Program
<?php $array = ["a"=>2, "b"=>5, "c"=>7, "e"=>9, "f"=>1, "g"=>0, "h"=>8, "i"=>3]; $chunks = array_chunk($array, 3); echo "The chunks are"; foreach ($chunks as $chunk) { echo "<br>"; print_r($chunk); } ?>
Output
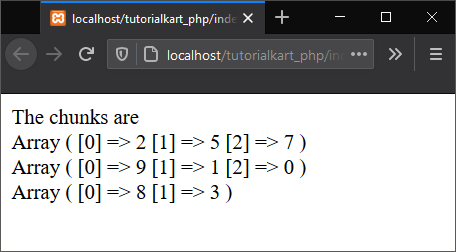
There are two observations that we can draw from this output. They are
- The keys in the chunk arrays are 0, 1, 2: which is not as our source array. This is because, by default array_chunk() does not preserve the keys.
- The last chunk has only two elements which is less than the given chunk size. This happens for the last chunk if the array does not have exact multiple of given chunk size.
2. Split array into chunks, but preserve the keys
In this example, we will take an associative array with key-value pairs, same as in the previous example, and then split into chunks of size 3, but preserve the keys by passing TRUE for preserve_keys argument.
PHP Program
<?php $array = ["a"=>2, "b"=>5, "c"=>7, "e"=>9, "f"=>1, "g"=>0, "h"=>8, "i"=>3]; $chunks = array_chunk($array, 3, TRUE); echo "The chunks are"; foreach ($chunks as $chunk) { echo "<br>"; print_r($chunk); } ?>
Output
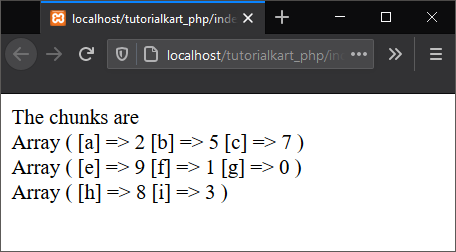
The keys in the chunks are preserved from the source array.
3. Warning: array_chunk(): Size parameter expected to be greater than 0
The chunk size has to be greater than zero. If it is less than one, array_chunk() throws a warning and returns NULL.
In the following example, we will call array_chunk() function with size = 0.
PHP Program
<?php $array = ["a"=>2, "b"=>5, "c"=>7, "e"=>9, "f"=>1, "g"=>0, "h"=>8, "i"=>3]; $chunks = array_chunk($array, 0); ?>
Output
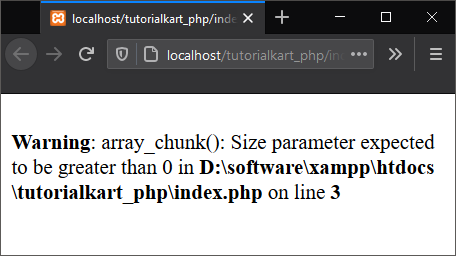
Conclusion
In this PHP Tutorial, we learned how to change the case of all keys in given array using PHP Array array_change_key_case() function.