In this tutorial, you shall learn elseif statement in PHP, its syntax, working, and how to and when to use elseif statement with example programs.
ElseIf Statement
PHP ElseIf (If ElseIf) statement is kind of an extension to If-Else statement in terms of number of conditions and number of code blocks.
In If-Else statement we have only one condition to check, but, in Else-If statement, we can have more than one conditions. And these conditions can be prioritised. The priority of the conditions decreases from top to bottom of the Else-If statement.
Consider the following pseudo code for If ElseIf statement with three conditions.
if ( condition_1 ) { //block-1 } elseif ( condition_2 ) { //block-2 } elseif (condition_3 ) { //block-3 }
The flow of execution is from top to bottom.
First condition_1
is evaluated. If it evaluates to true
, the block-1
is executed, and out of the if-elseif statement. If the condition_1
evaluates to false
, the program control moves to the next elseif line.
If the program control has come to evaluating condition_2
, and if the condition_2
evaluates to true
, then block-2
executes, and out of the if-elseif statement. But if the condition_2
is false
, then the program control moves to the next elseif line (if there is one), and so on.
If we want some code to run when none of the conditions evaluate to true
, we can write an optional else block at the end, as shown in the following.
if ( condition_1 ) { //block-1 } elseif ( condition_2 ) { //block-2 } elseif (condition_3 ) { //block-3 } else { //default block }
Examples
1. Find largest of three numbers using if-elseif statement.
In the following program, we use if-elseif statement to find the largest of the three numbers a
, b
, and c
.
The first condition would be to check if a
is greater than b
and c
.
The second condition would be to check if b
is greater than c
.
At last, we can have an else block, to declare that c
is the largest if none of the above two conditions is true
.
PHP Program
<?php $a = 8; $b = 42; $c = 6; if ( $a > $b && $a > $c ) { echo 'a is the largest.'; } elseif ( $b > $c ) { echo 'b is the largest.'; } else { echo 'c is the largest.'; } ?>
Output
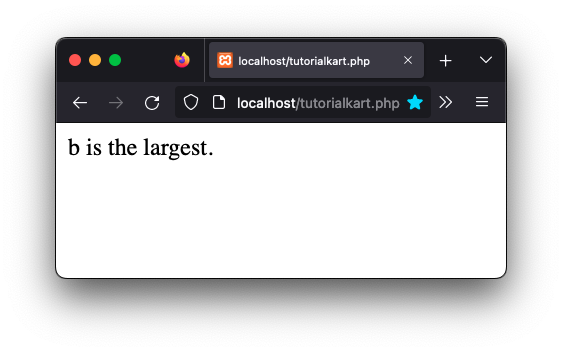
Conclusion
In this PHP Tutorial, we learned about elseif statement, and how to use elseif statement in a PHP program, with the help of examples.