In this tutorial, you shall learn about PHP array_diff() function which finds the difference of an array against other arrays, with syntax and examples.
PHP array_diff() Function
The PHP Array array_diff() function compares the values of an array against one or more arrays, and returns the differences.
This function compares the values of an array, say array1, against one or more arrays, say, array2, array3, etc., and returns an array that contains the entries that are present in array1 but not present in array2, array3, etc.
Please note that array_diff() compares only the values and find the differences between arrays. For indexed arrays or associative arrays, array_diff() does not consider index/key for comparison.
For example, the items “a”=>”apple”, “A”=>”apple”, 1=>”apple”, “apple” are all equal with respect to array_diff() function.
Syntax of array_diff()
The syntax of array_diff() function is
array_diff( array1, array2, array3, ...)
where
Parameter | Description |
---|---|
array1 | [mandatory] The array to compare items from. |
array2 | [mandatory] An array to compare against. |
array3,… | [optional] More arrays to compare against. |
Function Return Value
array_diff() returns an array containing the entries from array1 that are not present in any of the other arrays: array2, array3, …
Examples
1. Difference between Two Arrays
In this example, we will take two arrays: $array1 and $array2. We will use array_diff() function and compare $array1 against $array2. The function returns elements present in $array1, but not in $array2.
PHP Program
<?php $array1 = array("apple", "banana", "mango"); $array2 = array("banana", "mango"); $result = array_diff($array1,$array2); print_r($result); ?>
Output
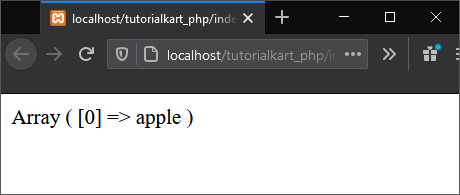
2. Difference between an array against two other arrays
In this example, we will take three arrays: $array1, $array2 and $array3. We will use array_diff() function and compare $array1 against $array2 and $array3. The function returns elements present in $array1, but not in $array2 and $array3 combined.
PHP Program
<?php $array1 = array("apple", "banana", "mango"); $array2 = array("fig", "mango"); $array3 = array("apple", "orange"); $result = array_diff($array1, $array2, $array3); print_r($result); ?>
Output
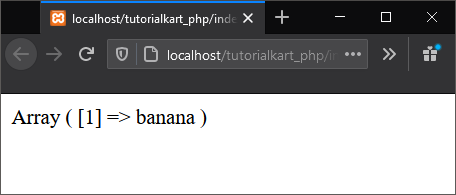
Conclusion
In this PHP Tutorial, we learned how to compare two arrays and find the differences in their values, using PHP Array array_diff() function.