In this tutorial, you shall learn about Classes in PHP, the syntax of a class, how to define a class with members(properties and functions), with the help of example programs.
Class
Class is one of the main aspects of object oriented programming. A class is used to define a custom type with specific properties and behaviour.
Syntax
The syntax to define a class is:
Start with class
keyword, followed by a class name, followed by an opening curly brace, then the properties and methods of the class, followed by a closing curly brace, as shown in the following.
class ClassName {
//properties
//methods
}
Rules for writing Class Name
- The class name must not be a PHP reserved word.
- The class name starts with a letter or underscore. Rest of the classname can be any number of letters, numbers, or underscores.
Example
In the following example, we define a class with class name Person
. It has two properties fullname
and age
, and one method printDetails()
.
<?php
class Person {
public $fullname;
public $age;
public function printDetails() {
echo "Name : " . $this->fullname . "<br>Age : " . $this->age;
}
}
?>
Now, let us create an object $person1
of this class type Person, set its properties, and call a method on this object.
PHP Program
<?php
class Person {
public $fullname;
public $age;
public function printDetails() {
echo "Name : " . $this->fullname . "<br>Age : " . $this->age;
}
}
//create object of type Person
$person1 = new Person();
//set properties of the object with values
$person1->fullname = "Mike Turner";
$person1->age = "24";
//call method on the object
$person1->printDetails();
?>
Output
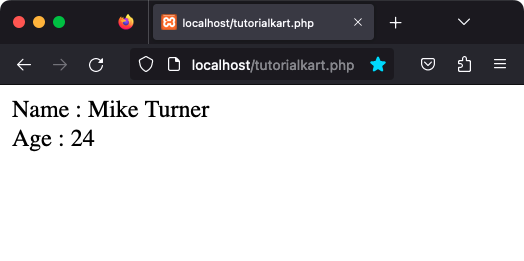
We can create any number of objects of type Person like $person1
and these objects will have the specified properties and behaviours defined in the class definition.
We will learn more about creating objects in our next tutorials.
Conclusion
In this PHP Tutorial, we learned about classes, and how to define a class using syntax and examples. In our next tutorials we will learn more about objects.