In this PHP tutorial, you shall learn how to alphabets in a string using ctype_alpha() function, with example programs.
PHP – Count only Alphabets in String
To count only alphabets in a string, iterate over the characters of the strings, and for each character increment your counter if the character is an alphabet. We can check if the character is an alphabet or not using ctype_alpha() built-in PHP function.
Examples
1. Count Alphabets in the string “Hello World!”
In this example, we will take a string “Hello World!” in a variable $string. Get the string length into $length using strlen() function. Then we will write a for loop to access each character of the string iteratively and for each character we will check if it is an alphabet. If it is an alphabet, we will increment the counter for number of alphabets in the string.
PHP Program
<?php
$string = "Hello World!";
$count = 0;
$length = strlen($string);
for ($i = 0; $i < $length; $i++) {
if( ctype_alpha($string[$i]) ) {
$count++;
}
}
echo $count;
?>
Output
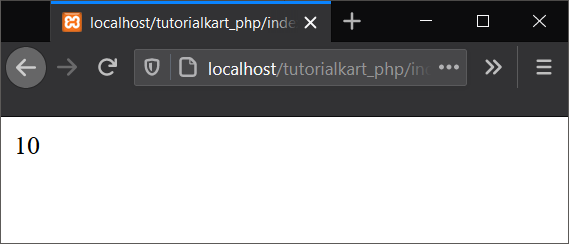
Conclusion
In this PHP Tutorial, we learned how to count total number of alphabets in a string using ctype_alpha() function.