In this tutorial, you shall learn about PHP array_replace() function which can update the values in this array with values from other array based on keys, with syntax and examples.
PHP array_replace() Function
The PHP array_replace() function replaces the values of an array (array1) with the values from other array(s) based on key/index.
Please note that, if you are working with indexed arrays, then the index of items will act as key.
Syntax of array_replace()
array_replace( array1, array2, array3, ...)
where
Parameter | Description |
---|---|
array1 | [mandatory] Specifies an array |
array2 | [optional] Specifies an array which will replace the values of array1 |
array3,… | [optional] Specifies more arrays to replace the values of array1 and array2, etc. Values from later arrays will overwrite the previous ones. |
If a key from array1 exists in array2, then value for this key in array1 will be replaced by the corresponding key’s value from array2.
If a key only exists in array1, and not in any other arrays, then its value will be left as it is.
If a key exist in array2 and not in array1, then this key-value pair will be inserted in array1.
If multiple arrays (array2, array3,…) are used, values from later arrays (say array3) will overwrite the previous ones (array2).
We will go through examples for each of these scenarios.
Function Return Value
array_replace() returns the replaced array. If any error occurs during function execution, it returns NULL.
Examples
1. Replace Values in Array – Key exists in array2
In this example, we will take an array (array1) with two key value pairs. The array2 will have a key-value pair whose key "b"
exists in array1. When we replace values of array1 with that of array2 using array_replace() function, the value for key "b"
should be replaced in array1 by array2.
PHP Program
<?php $array1 = array("a"=>"apple", "b"=>"banana"); $array2 = array("b"=>"berry"); $result = array_replace($array1, $array2); print_r($result); ?>
Output
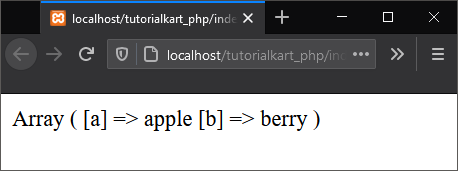
In addition to what happened to key "b"
, observe what happened to key "a"
. Since key "a"
is present only in array1, but not in array2, the value for key "a"
in array1 is left unchanged.
2. Replace Value in Array – Key exists in array2 but not in array1
If there exists a key which is present in array2, but not in array1, then this key-value pair will be inserted in array1.
PHP Program
<?php $array1 = array("a"=>"apple", "b"=>"banana"); $array2 = array("m"=>"mango"); $result = array_replace($array1, $array2); print_r($result); ?>
Output
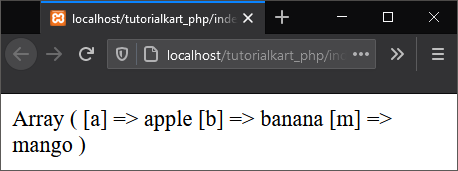
3. Replace Value in Array – Multiple Arrays
If we provide more than one array for replacement, then the values for the keys in later arrays will replace the value of key in array1.
In the following example, the key “b” is present in array1, array2 and array3. So, the value for key “b” in array1 will be replaced by the value corresponding to the key in array3, because array3 is the later one to array2.
PHP Program
<?php $array1 = array("a"=>"apple", "b"=>"banana"); $array2 = array("b"=>"berry"); $array3 = array("b"=>"bing-cherry"); $result = array_replace($array1, $array2, $array3); print_r($result); ?>
Output
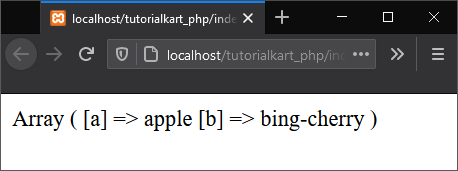
4. array_replace() with Indexed Arrays
If we take indexed arrays, the index of items in the array act as keys when used with array_replace() function.
In this example, we will take indexed arrays: array1 and arra2; and replace values in array1 with values in array2.
PHP Program
<?php $array1 = array("apple", "banana", "cherry"); $array2 = array("mango", "orange"); $result = array_replace($array1, $array2); print_r($array1); echo "<br>"; print_r($array2); echo "<br>Replaced array : <br>"; print_r($result); ?>
Output
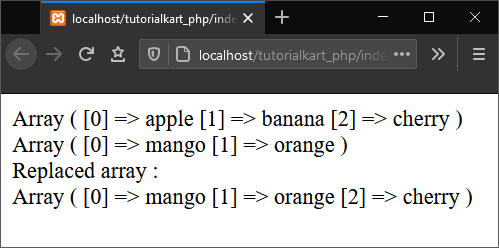
Conclusion
In this PHP Tutorial, we learned how to replace values in an array with values from other arrays based on keys, using PHP Array array_replace() function.