In this tutorial, you shall learn how to parse a JSON string in PHP using json_decode() function, with syntax and example programs.
PHP – Parse JSON String
To parse a JSON String in PHP, call json_decode()
function and pass the JSON string. This function can take other optional parameters also that effect the return value or parsing.
Syntax
The syntax of json_decode()
function is
json_decode(string, associative, depth, flags)
where
Parameter | Description |
---|---|
string | [Required] JSON string to be parsed or decoded. |
associative | [Optional] Boolean Value. If true, the returned object will be converted into an associate array. If false (default value), an object is returned. |
depth | [Optional] Integer. Specifies the depth of recursion. Default value = 512. |
flags | [Optional] Bitmask: JSON_BIGINT_AS_STRING, JSON_INVALID_UTF8_IGNORE, JSON_INVALID_UTF8_SUBSTITUTE, JSON_OBJECT_AS_ARRAY, JSON_THROW_ON_ERROR |
Generally, the first two parameters are sufficient in most use cases while parsing a JSON string. The other two parameters are used only in some special scenarios.
Examples
1. Parse JSON array string into a PHP array
In this example, we will take a JSON string and parse it using json_decode()
function with the default optional parameters. We shall display the object returned by json_decode()
in the output.
PHP Program
<?php
$jsonStr = '{"apple": 25, "banana": 41, "cherry": 36}';
$output = array(json_decode($jsonStr));
var_dump($output);
?>
Output
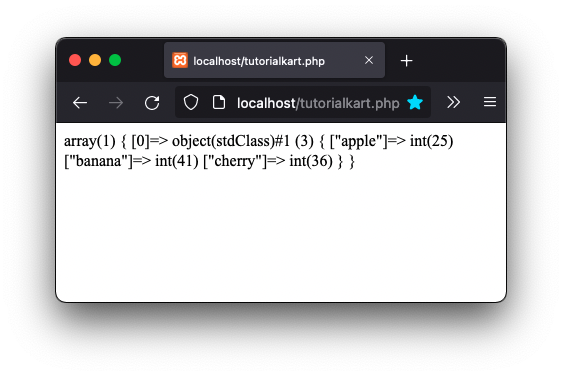
2. Access values in decoded JSON object
After decoding into PHP object, we can access the values using keys as shown in the following program.
PHP Program
<?php
$jsonStr = '{"apple": 25, "banana": 41, "cherry": 36}';
$output = json_decode($jsonStr);
echo $output->apple;
echo '<br>';
echo $output->banana;
echo '<br>';
echo $output->cherry;
echo '<br>';
?>
Output
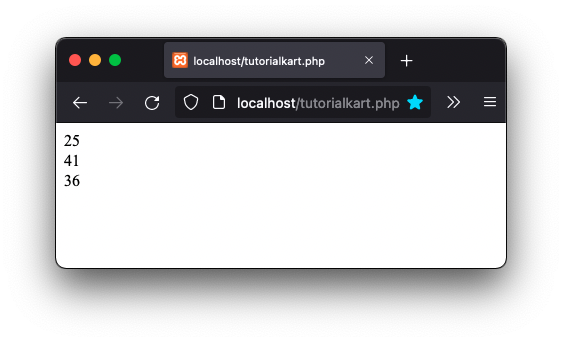
3. Decode JSON string into an array
We can directly decode the JSON string into an associative array by passing true for the second argument. And then we can use indexing technique to access the values for corresponding keys.
PHP Program
<?php
$jsonStr = '{"apple": 25, "banana": 41, "cherry": 36}';
$output = json_decode($jsonStr, true);
echo $output['apple'];
echo '<br>';
echo $output['banana'];
echo '<br>';
echo $output['cherry'];
echo '<br>';
?>
Output
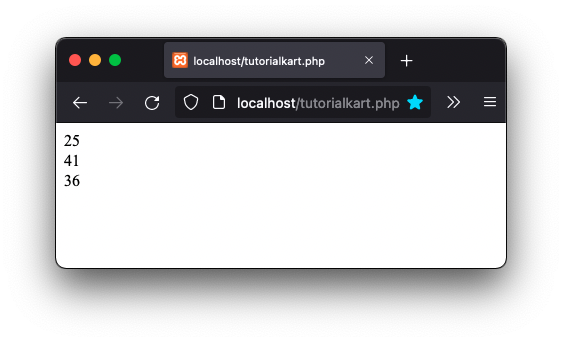
Conclusion
In this PHP Tutorial, we learned how to parse a JSON string, using json_decode()
function.