In this tutorial, you shall learn how to convert an integer value to a floating point value in PHP, using typecasting, or floatval() function, with the help of example programs.
PHP – Convert Integer to Float
To convert an integer type value to a floating point type value in PHP, you can use Type Casting technique or PHP built-in function floatval().
Method 1: Type Casting
To convert integer to float using Type Casting, provide the literal (float)
along with parenthesis before the integer value. This expression returns a float value. We can store it in a variable or use it as a part of another expression.
The syntax to type-cast integer to floating point value is
$float_value = (float) $int_value;
In the following program, we take an integer value in $n
, convert this integer value to a float value using type-casting, and store the result in $f
.
PHP Program
<?php
$n = 268;
$f = (float) $n;
echo "float value : " . $f;
?>
Output
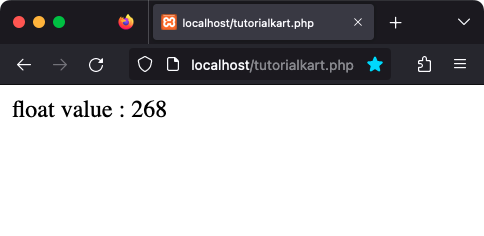
Method 2: floatval()
To convert integer value to floating point value using PHP built-in function floatval(), pass the integer value as argument to the function. The function returns a floating point value created from the given argument.
The syntax to use floatval() to convert integer number to floating point number is
$float_value = floatval( $integer_value );
In the following program, we take an integer value in $n, convert it to float and store the result in $f
.
PHP Program
<?php
$n = 268;
$f = floatval($n);
echo "float value : " . $f;
?>
Output
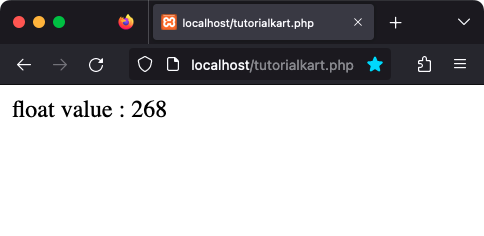
Conclusion
In this PHP Tutorial, we learned how to convert an integer number to a floating point number using type-casting or floatval() function.