In this PHP tutorial, you will learn how to use AND operator in If-statement condition, and some example scenarios.
PHP If AND
PHP If condition can be compound condition. So, we can join multiple simple conditions with logical AND operator and use it as condition for PHP If statement.
If statement with AND operator in the condition
The typical usage of an If-statement with AND logical operator is
if ( condition_1 && condition_2 ) {
//if-block statement(s)
}
where
- condition_1 and condition_2 can be simple conditional expressions or compound conditional expressions.
&&
is the logical AND operator in PHP. It takes two operands: condition_1 and condition_2.
Since we are using AND operator to combine the condition, PHP executes if-block only if both condition_1 and condition_2 are true. If any of the conditions evaluate to false, PHP does not execute if-block statement(s).
Examples
1. Check if a is 2 and b is 5
In this example, we will write an if statement with compound condition. The compound condition contains two simple conditions and these are joined by AND logical operator.
PHP Program
<?php
$a = 2;
$b = 5;
if ( ( $a == 2 ) && ( $b == 5 ) ) {
echo "a is 2 and b is 5.";
}
?>
Output
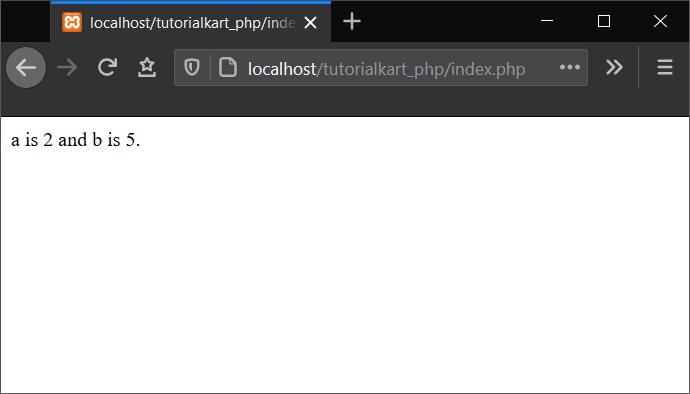
2. Check if given string starts with “a” and ends with “e”.
In this example we use AND operator to join two conditions. The first condition is that the string should start with "a"
and the second condition is that the string should end with "e"
.
PHP Program
<?php
$name = "apple";
if ( str_starts_with($name, "a") && str_ends_with($name, "e") ) {
echo "$name starts with a and ends with e.";
}
?>
Output
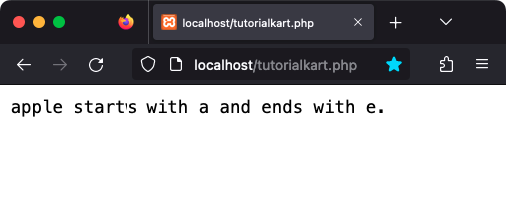
Conclusion
In this PHP Tutorial, we learned how to write PHP If statement with AND logical operator.