In this tutorial, you shall learn about Arithmetic Operators in PHP, different Arithmetic Operators available in PHP, their symbols, and how to use them in PHP programs, with examples.
Arithmetic Operators
Arithmetic Operators are used to perform basic mathematical arithmetic operators like addition, subtraction, multiplication, etc.
Arithmetic Operators Table
The following table lists out all the arithmetic operators in PHP programming.
Operator | Symbol | Example | Description |
---|---|---|---|
Addition | + | x + y | Returns addition of x and y . |
Subtraction | – | x - y | Returns the subtraction of y from x . |
Multiplication | * | x * y | Returns the multiplication of x and y . |
Division | / | x / y | Returns the quotient of the result of division of x by y . |
Modulus | % | x % y | Returns the reminder of division of x by y . Known as modular division. |
Exponent | ** | x ** y | Returns x raised to the power y . |
Example
In the following program, we will take values in variables $x
and $y
, and perform arithmetic operations on these values using PHP Arithmetic Operators.
PHP Program
<?php $x = 5; $y = 2; $addition = $x + $y; $subtraction = $x - $y; $multiplication = $x * $y; $division = $x / $y; $modulus = $x % $y; $exponentiation = $x ** $y; echo "x = $x" . "<br>"; echo "y = $y" . "<br>"; echo "x + y = $addition" . "<br>"; echo "x - y = $subtraction" . "<br>"; echo "x * y = $multiplication" . "<br>"; echo "x / y = $division" . "<br>"; echo "x % y = $modulus" . "<br>"; echo "x ** y = $exponentiation"; ?>
Output
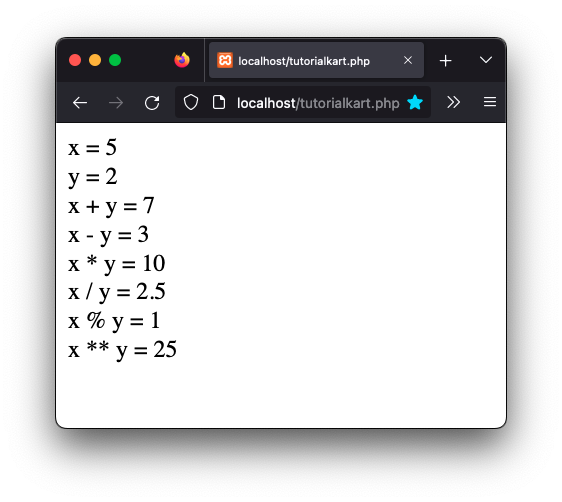
Arithmetic Operators Tutorials
The following tutorials cover each of the Arithmetic Operators in PHP, in detail with examples.
- PHP Addition PHP Tutorial on how to use Addition Operator to add two numbers.
- PHP Subtraction PHP Tutorial on how to use Subtraction Operator to subtract a number from another.
- PHP Multiplication PHP Tutorial on how to use Multiplication Operator to find the product of two numbers.
- PHP Division PHP Tutorial on how to use Division Operator to find the quotient of division of given two numbers.
- PHP Modulus PHP Tutorial on how to use Modulo Operator to find the remainder of integer division of given two numbers.
- PHP Exponentiation PHP Tutorial on how to use Increment Operator to increase the value in a variable by one.
Conclusion
In this PHP Tutorial, we learned about all the Arithmetic Operators in PHP programming, with examples.