In this tutorial, you shall learn how to check if a string contains at least one match for a given regular expression in PHP using preg_match() function, with the help of example programs.
PHP – Check if string has a match for a regular expression
To check if a string contains at least one match for a given regular expression in PHP, we can use preg_match() function.
Call the preg_match() function and pass the pattern, and input string as arguments.
preg_match($pattern, $str)
If the string $str
contains a match for the regular expression $pattern
, then the function returns 1
. If there is no match, then the function returns 0
. Or else in case of any error, a boolean value of false is returned.
Examples
1. Check if string has a match for “fox”, case insensitive
In this example, we take a test string $str
, and check if the string contains the "fox"
and the matching has to happen insensitive to case. The pattern would be "/fox/i"
.
PHP Program
<?php $str = "The quick brown fox jumps over the lazy dog."; $pattern = "/fox/i"; if(preg_match($pattern, $str)) { echo "There is a match."; } else { echo "There is no match."; } ?>
Output
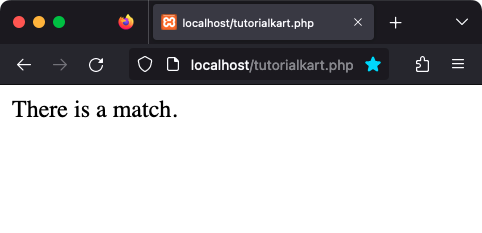
Now, let us take another test string and check if it has a match for the "fox"
.
PHP Program
<?php $str = "The cat in the hat sat on the mat."; $pattern = "/fox/i"; if(preg_match($pattern, $str)) { echo "There is a match."; } else { echo "There is no match."; } ?>
Output
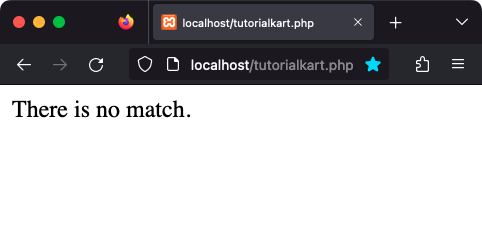
Reference to tutorials related to program
Conclusion
In this PHP Tutorial, we learned how to check if the string has a match for a given pattern, or not, using preg_match() function, with the help of examples.