In this tutorial, you shall learn about PHP array_keys() function which can get the keys in an array, with syntax and examples.
PHP array_keys() Function
The PHP array_keys() function returns keys of a given array. You can also specify a value, so that array_keys() returns only those keys whose value matched this value.
array_keys() function returns keys as an indexed array.
Syntax of array_keys()
The syntax of array_keys() function is
array_keys( array, value, strict)
where
Parameter | Description |
---|---|
array | [mandatory] The array which we have to work on. |
value | [optional] The keys in the array, which has this value only, will be returned. |
strict | [optional] Used with the value parameter. If strict is TRUE, then the type of value is also matched. If strict is FALSE, then the type of value is not considered for comparison of values. |
Function Return Value
array_keys() returns an array containing the keys of given array.
Examples
1. Get keys in the given array
In this example, we will take an array with key-value pairs. We will call array_keys() function with the array provided as argument, to get all the keys in the array, and then print the keys array.
PHP Program
<?php
$array1 = array("a"=>21, "b"=>54, "m"=>35, "k"=>66);
$keys = array_keys($array1);
print_r($keys);
?>
Output
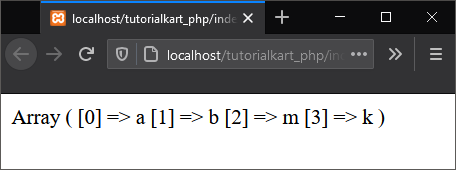
2. Get keys of an Indexed Array
In our previous example, we have used an associative array. In this example, we will take an indexed array. In indexed array, indexes are the keys.
PHP Program
<?php
$array1 = array(21, 54, 7=>21, 66);
$keys = array_keys($array1);
print_r($keys);
?>
Output
array_keys() just returns the indexes of all the values as an array.
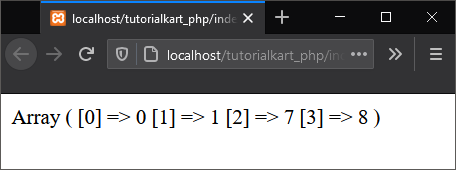
3. Get keys of an Array with specified value
In this example, we will take an array with key-value pairs. We will use array_keys() function to fetch only those values with a specific value.
PHP Program
<?php
$array1 = array("a"=>21, "b"=>54, "m"=>21, "k"=>66, "z"=>21);
$value = 21;
$keys = array_keys($array1, $value);
print_r($keys);
?>
Output
The value 21 matches with values of three keys: a
, m
, z
.
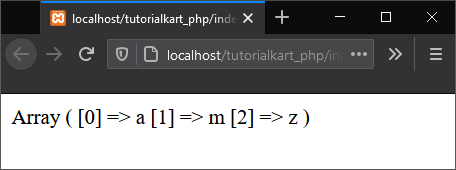
The same explanation holds for an indexed array.
4. Pass the parameter strict = TRUE to array_keys()
In this example, we will provide TRUE
as argument for the parameter strict
.
PHP Program
<?php
$array1 = array("a"=>21, "b"=>54, "m"=>"21", "k"=>66, "z"=>21);
$value = 21;
$strict = TRUE;
$keys = array_keys($array1, $value, $strict);
print_r($keys)
?>
Output
The value 21 matches strictly matches with values of two keys: "a"
, "z"
. The type of value 21 does not match the type of value corresponding to the key "m"
.
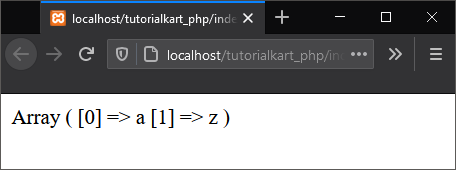
5. Pass the parameter strict = FALSE for array_keys()
In this example, we will provide FALSE
as argument for the parameter strict
.
PHP Program
<?php
$array1 = array("a"=>21, "b"=>54, "m"=>"21", "k"=>66, "z"=>21);
$value = 21;
$strict = FALSE;
$keys = array_keys($array1, $value, $strict);
print_r($keys)
?>
Output
If we do not consider the datatype of the values, then the values corresponding to the keys: "a"
, "z"
and "m"
matches.
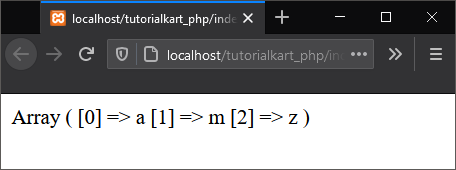
If we take an indexed array, we will get the indexes of those items whose value match.
PHP Program
<?php
$array1 = array(21, 54, "21", 66, 21);
$value = 21;
$strict = FALSE;
$keys = array_keys($array1, $value, $strict);
print_r($keys)
?>
Output
Since strict is FALSE, the type of value is not considered for comparison. So, 0, 2 and 4 are the indexes that match the given value.
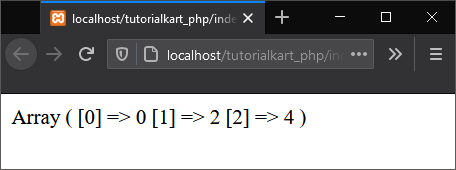
Conclusion
In this PHP Tutorial, we learned how to get keys of given array, using PHP Array array_keys() function.