In this tutorial, you shall learn how to find the difference between two given dates in PHP using date_diff() function, with example programs.
PHP – Difference between Two Dates
To calculate the difference between two dates in PHP, call date_diff()
date/time function, and pass the two dates as argument to it.
date_diff()
function returns a DateInterval
object, or FALSE
if calculating the difference is not successful. DateInterval object has properties $y
for year, $m
for months, $d
for days, $h
for hours, $i
for minutes, $s
for seconds, $f
for microseconds, $invert
to represent if the interval is negative or not.
Examples
1. Find the difference between two dates: date1 – date2
In the following program, we shall take two dates in $date1
and $date2
and find their difference.
PHP Program
<?php
$date1 = date_create( "2022-01-15 12:23:17" );
$date2 = date_create( "2022-02-22 11:05:25" );
$diff = date_diff( $date1, $date2 );
print_r( $diff );
?>
Output
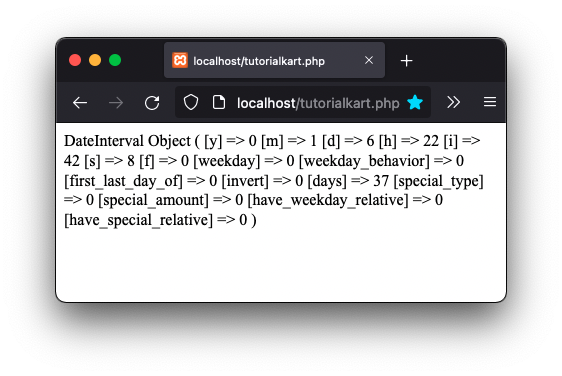
We can access the individual properties of the DateInterval object, as shown in the following program.
PHP Program
<?php
$date1 = date_create( "2022-01-15 12:23:17" );
$date2 = date_create( "2022-02-22 11:05:25" );
$diff = date_diff( $date1, $date2 );
echo "Years : ", $diff -> y;
echo "<br>";
echo "Months : ", $diff -> m;
echo "<br>";
echo "Days : ", $diff -> d;
echo "<br>";
echo "Hours : ", $diff -> h;
echo "<br>";
echo "Minutes : ", $diff -> i;
echo "<br>";
echo "Seconds : ", $diff -> s;
echo "<br>";
?>
Output
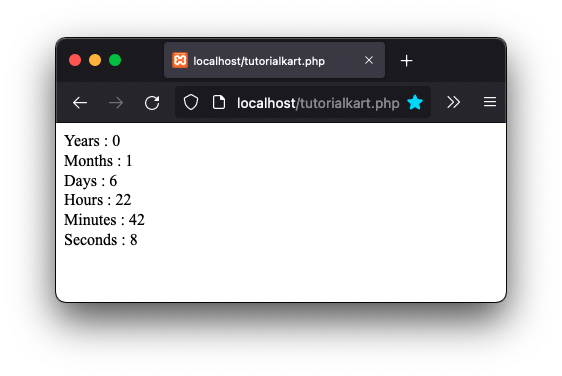
Conclusion
In this PHP Tutorial, we learned how to find the difference between two dates in PHP, using date_diff()
function.