In this tutorial, you shall learn how to replace all occurrences of a search string in a string with a replacement string in PHP using str_replace() function, with the help of example programs.
PHP – Replace String
To replace all occurrences of a search string in a string with a replacement string in PHP, call str_replace() function and pass the search string, replacement string, and original string as arguments.
Syntax
The syntax of str_replace() function to replace all occurrences of a search string $search
with a replacement string $replacement
in the original string $subject
is
str_replace($search, $replacement, $subject)
The above function call returns a new string with all the search string occurrences replaced with the replacement string. The original string remains unchanged.
Examples (2)
1. Replace operation when search string is present in the string
In this example, we take an input string "apple banana apple mango"
and replace the search string "apple"
with the replacement string "cherry"
in this input string.
PHP Program
<?php $input_string = "apple banana apple mango"; $search = "apple"; $replacement = "cherry"; $output_string = str_replace($search, $replacement, $input_string); echo "<html><pre>"; echo "Input String : " . $input_string; echo "<br>Output String : " . $output_string; echo "</pre></html>"; ?>
Output
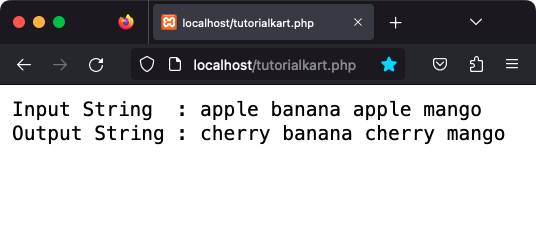
Reference tutorials for this program
2. Replace operation when search string is not present in the string
In this example, we take an input string "apple banana apple mango"
and replace the search string "guava"
with the replacement string "cherry"
in this input string.
Since the search string is not present in the original string, the output string from str_replace() function would be similar to the input original string.
PHP Program
<?php $input_string = "apple banana apple mango"; $search = "guava"; $replacement = "cherry"; $output_string = str_replace($search, $replacement, $input_string); echo "<html><pre>"; echo "Input String : " . $input_string; echo "<br>Output String : " . $output_string; echo "</pre></html>"; ?>
Output
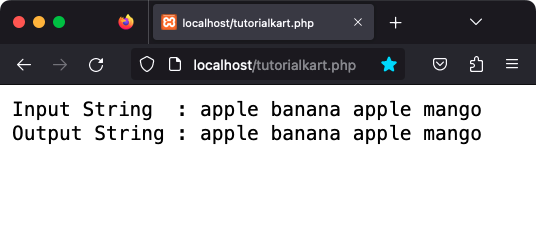
Reference tutorials for this program
Conclusion
In this PHP Tutorial, we learned how to replace all occurrences a search string with a replacement string, using str_replace() function.