In this tutorial, you shall learn how to check if specific element is present in given array in PHP using in_array() function, with the help of example programs.
PHP – Check if specific element is present in array
To check if specific element in present in an array or not in PHP, we can use in_array()
function. in_array()
function takes an element and array as arguments, and returns a boolean value representing if the element is present in array. If in_array()
returns true, then the specific element is present in the array.
The syntax of the condition to check if element value is present in the array arr
is
in_array($value, $arr)
Examples
1. Check if element “mango” is present in array
In this example, we will take an array arr
with some string elements in it, and programmatically check if this array arr
contains the element "mango"
, using in_array()
function.
PHP Program
<?php $arr = ["apple", "banana", "mango", "cherry"]; $value = "mango"; if ( in_array($value, $arr) ) { echo "The element is in array."; } else { echo "The element is not in array."; } ?>
Output
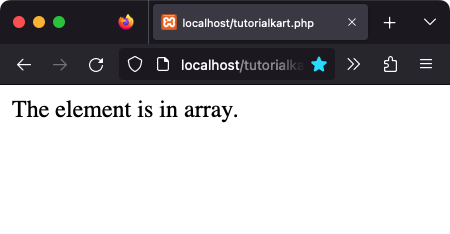
2. Check if element “avocado” is present in array
In this example, we will take an array arr
with some elements in it, and programmatically check if this array arr
is empty or not using count()
function.
PHP Program
<?php $arr = ["apple", "banana", "mango", "cherry"]; $value = "avocado"; if ( in_array($value, $arr) ) { echo "The element is in array."; } else { echo "The element is not in array."; } ?>
Output
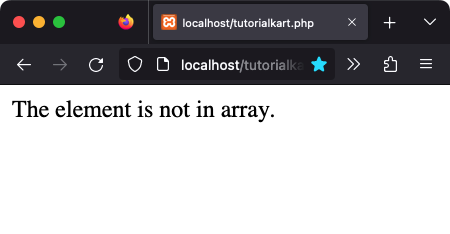
Conclusion
In this PHP Tutorial, we learned how to check if specific element is present in an array or not using in_array()
function.