In this PHP tutorial, you shall learn how to check if given string ends with forward slash character using strcmp() function, with example programs.
PHP – Check if String Ends with Slash
To check if string ends with slash character, get the last character of the string using indexing and check if that is slash.
We can use strcmp() to compare of the last character of the given string with slash (/). The syntax of condition using strcmp() to check if last character is slash is
strcmp($lastchar, "/") === 0
strcmp() returns true is last character is slash, else it returns false.
Examples
1. Check if given URL string ends with slash character
In this example, we will take a string in a variable, get the last character of it, and compare with forward slash (/) using strcmp() built-in PHP function.
PHP Program
<?php $string = "https://www.tutorialkart.com/"; $lastchar = $string[-1]; if ( strcmp($lastchar, "/") === 0 ) { echo "\"{$string}\" ends with \"{$lastchar}\"."; } else { echo "\"{$string}\" does not end with \"{$lastchar}\"."; } ?>
Output
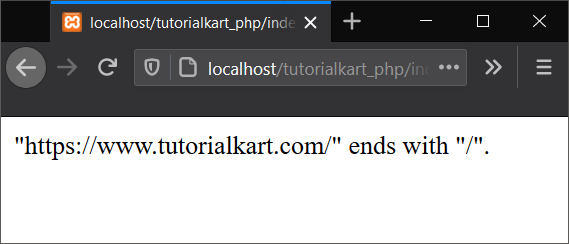
Conclusion
In this PHP Tutorial, we learned how to check if a string ends with slash character, using PHP built-in function strcmp().