In this tutorial, you shall learn how to din the index of a specific key in an array in PHP using array_search() function, with example programs.
PHP – Find index of a key in array
To find the index of a key in an array in PHP, you can use array_search() function.
Call array_search() function, and pass the key, and array keys as arguments. The function returns an integer value representing the index of the key in the array.
The following function call finds the index of the key $key
in array $arr
.
array_search($key, array_keys($arr))
If given key is not present in the array, the function returns a boolean value of false.
We have used array_keys() function to get the keys of the associative array.
Examples
1. Find index of key ‘das’ in array
In the following program, we take an associative array $arr
, and find the index of the key 'das'
in the array using array_search() function.
PHP Program
<?php $arr = array( 'foo' => 10, 'bar' => 20, 'das' => 30, 'qux' => 40, 'par' => 50, 'saz' => 60, ); $key = 'das'; //get index of the key in the array $index = array_search($key, array_keys($arr)); if ($index) { print_r("Index of given key : {$index}"); } else { print_r("Key is not present in the array."); } ?>
Output
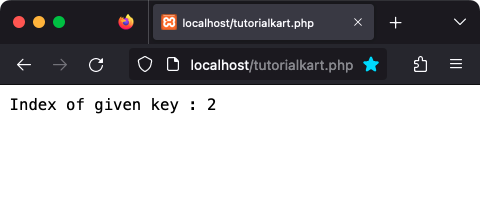
2. Find index of key ‘apple’ in array
In the following program, we are trying to find the index of a key which is not present in the array. In this scenario, the function array_search() returns false, and as per the program, the else block must execute.
PHP Program
<pre> <?php $arr = array( 'foo' => 10, 'bar' => 20, 'das' => 30, 'qux' => 40, 'par' => 50, 'saz' => 60, ); $key = 'apple'; //get index of the key in the array $index = array_search($key, array_keys($arr)); if ($index) { print_r("Index of given key : {$index}"); } else { print_r("Key is not present in the array."); } ?> </pre>
Output
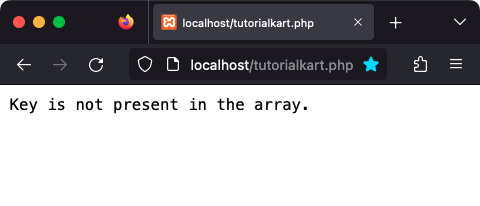
Conclusion
In this PHP Tutorial, we learned how to find the index of a key in an array, using array_search() function.