In this tutorial, you shall learn about PHP array_product() function which can find the product of all elements in an array, with syntax and examples.
PHP array_product() Function
The PHP array_product() function computes the product of numbers in an array and returns the result.
The array should contain only numeric datatypes: int, float. If array contains values of any other datatypes, then array_product() returns 0.
Syntax of array_product()
The syntax of array_product() function is
array_product( array)
where
Parameter | Description |
---|---|
array | [mandatory] Array whose elements’ product has to be computed. |
Function Return Value
array_product() returns the product as an integer or float
Examples
1. Product of numbers in an array
In this example, we will take an array with three numbers. We will pass this array as argument to array_product() function. The function computes the product of the numbers in the array and returns the result.
PHP Program
<?php $array1 = array(5, 7, 2); $result = array_product($array1); print_r($array1); echo "<br>Product is: "; echo $result; ?>
Output
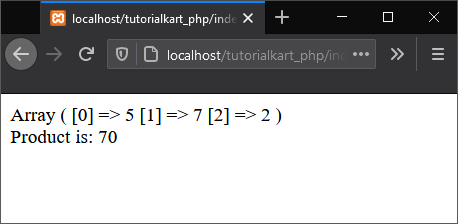
2. Product of Floating Point Numbers in Array
In this example, we will take an array with floating point numbers. We will find the product of these numbers in the array using array_product() function.
PHP Program
<?php $array1 = array(5.8, 2.2, 4.8); $result = array_product($array1); print_r($array1); echo "<br>Product is: "; echo $result; ?>
Output
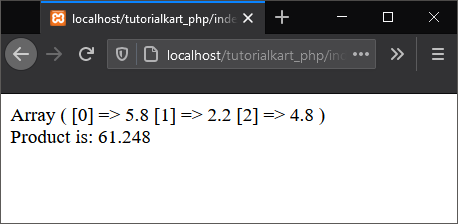
3. Product of Items in Array with Strings
In this example, we will take an array with numbers and strings. If there is even one value of type other than numeric datatypes, then array_product() function returns 0.
PHP Program
<?php $array1 = array(5, 2, "apple"); $result = array_product($array1); print_r($array1); echo "<br>Product is: "; echo $result; ?>
Output
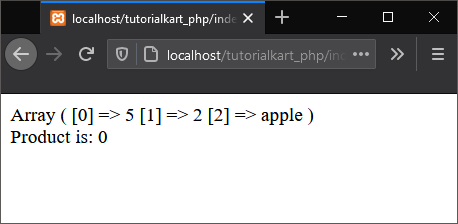
Conclusion
In this PHP Tutorial, we learned how to find the product of numbers in array, using PHP Array array_product() function.