In this tutorial, you shall learn how to about constructors in a class in PHP, and how to define a class constructor, with the help of example programs.
PHP – Class Constructor
A constructor in a class is used to initialise the properties of the class objects.
To define a constructor for a class in PHP, define __constructor() method in the class, just like any other function in a class, as shown in the following syntax.
class ClassName() { function __construct(parameters) { //constructor body } }
function
is keyword__construct
is the function name, and is fixed for a constructor in classparameters
: comma separated parameters that this constructor can accept as arguments.
Example
In our previous tutorials in PHP classes and objects series, we have given an example class Person
. We shall continue with this class Person
to demonstrate how to define a constructor for a class.
Now, let us define a constructor for the class Person
. And create an object with arguments passed for the constructor.
PHP Program
<?php class Person { protected string $fullname; protected int $age; public function __construct(string $fullname = null, int $age = null) { $this->fullname = $fullname; $this->age = $age; } public function printDetails() { echo "Name : " . $this->fullname . "<br>Age : " . $this->age; } } //create object of type Person with arguments passed for constructor $person1 = new Person("Mike Turner", 24); //call method on the object $person1->printDetails(); ?>
Output
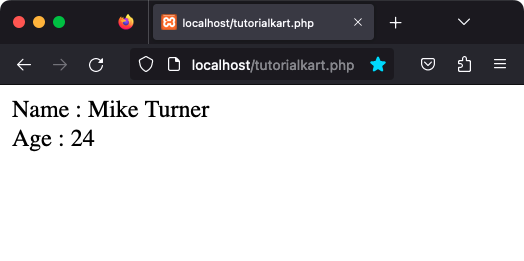
Conclusion
In this PHP Tutorial, we learned how to define a constructor for a class, with examples.