In this tutorial, you shall learn how to define a catch block in a try-catch statement in PHP to catch multiple exceptions in a single catch block, with the help of example programs.
PHP – Catch multiple exceptions in a single catch block
In PHP try catch statement, we can define a catch block to handle more than one exception type.
Syntax
The syntax of try catch statement with a single catch block handling multiple exception types is
try {
//code
} catch (Exception1 | Exception2 | Exception3 $e) {
//code
}
Replace Exception1
, Exception2
, Exception3
, .., with the type of exceptions that the try block can throw.
Please note that the exceptions or separated by Logical OR |
operator.
Example
In the following example, we have some code in try block that throws user defined Exceptions: NumberTooLargeException
or NumberTooSmallException
based on the value of $x
. We write a single catch block that can catch these two different exceptions.
PHP Program
<?php
class NumberTooLargeException extends Exception { }
class NumberTooSmallException extends Exception { }
try {
$x = 2;
if ($x < 10) {
throw new NumberTooSmallException("The given number is too small.");
} elseif ($x > 90) {
throw new NumberTooLargeException("The given number is too large.");
} else {
echo "x is within the desired range.";
}
} catch (NumberTooLargeException | NumberTooSmallException $e) {
//handle exception
echo $e->getMessage();
echo "<br>Number not in desired range.";
}
?>
Output
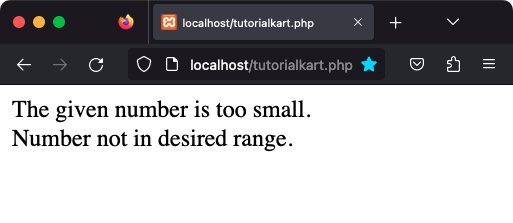
Conclusion
In this PHP Tutorial, we learned how to write a single catch block that can handle multiple exception types in a try-catch statement.