In this PHP tutorial, you shall learn how to check if given string is a valid floating point number, with example programs.
PHP – Check if String is Float
To check if string is a floating point number, typecast string to float. Convert this float to string and then check if the given string is equal to this converted string.
Typecasting from string to float will return a floating value. If type casting did not discard any characters while conversion from string to float, then the string equivalent of the float value should equal the given string.
The syntax to check if a string is float using is_float() is
$float_value = (float) $string; $is_string_float = ( strval($float_value) == $string )
In the above code snippet, strval() function converts float to string.
Examples
1. Check if given string is a floating value
In this example, we will take a string, whose value represents a floating point number, and verify programmatically if the string is a float or not using type conversion and equal to operator.
PHP Program
<?php $string = "3.14"; $float_value = (float) $string; if ( strval($float_value) == $string ) { echo "The string is a float value."; } else { echo "The string is not a float value."; } ?>
Output
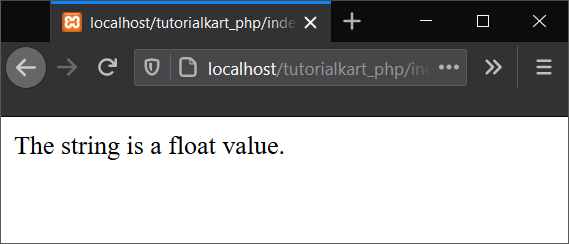
If you provide an integer, is_float() returns false.
2. Negative Scenario – String contains characters other than those allowed for a floating point number
In the following example, we will take a string which contains both digits and alphabets. We will check programmatically, if this string value can pass our test.
PHP Program
<?php $string = "414adsf"; $float_value = (float) $string; if ( strval($float_value) == $string ) { echo "The string is a float value."; } else { echo "The string is not a float value."; } ?>
Output
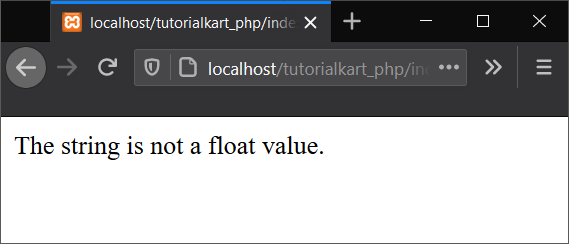
Conclusion
In this PHP Tutorial, we learned how to check if a string is floating point number or not.