In this tutorial, you shall learn how to split a given string by a specific delimiter string in PHP using explode() function, with syntax and example programs.
PHP – Split String by Delimiter/Separator
To split a string into parts using delimiter or separator string in PHP, use explode()
function. The explode()
function returns an array containing words as elements of the array.
explode()
function takes the delimiter/separator string, and the original string as arguments, and returns an array of strings obtained after splitting the given string using separator string.
Syntax
The syntax of explode()
function with the input string, and separator string as arguments is
explode(string $separator, string $input)
Examples
1. Split string by a separator string ‘xyz’
In this example, we will take an input string 'applexyzbananaxyzcherryxyzmango'
with all the parts separated by a separator string 'xyz'
. We split this string into an array of strings, where the input string is split at the delimiter string locations.
PHP Program
<?php
$input = 'applexyzbananaxyzcherryxyzmango';
$separator = 'xyz';
$output = explode($separator, $input);
foreach ($output as $x) {
echo $x;
echo '<br>';
}
?>
Output
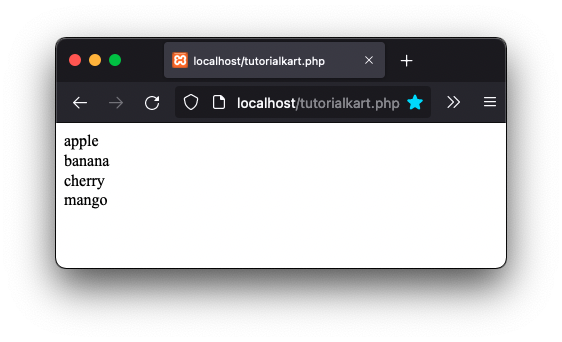
2. Split given string by a character separator
In this example, we will take an input string with all the parts separated by a character '-'
. We split this string into an array of strings, where the input string is split at the delimiter string locations.
PHP Program
<?php
$input = 'apple-banana-cherry-mango';
$separator = '-';
$output = explode($separator, $input);
foreach ($output as $x) {
echo $x;
echo '<br>';
}
?>
Output
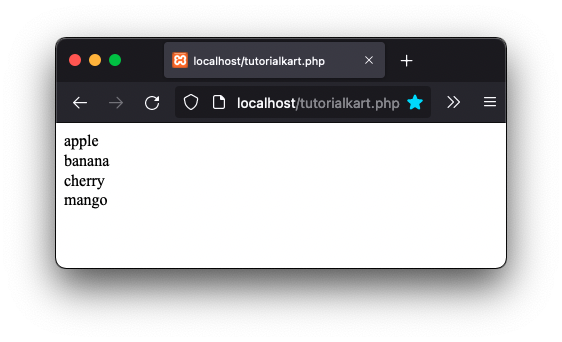
Conclusion
In this PHP Tutorial, we learned how to split a string into parts by delimiter/separator string, using explode()
function.