In this PHP tutorial, you shall learn how to check if given two strings are equal while ignoring the case of characters in the string using strcasecmp() function, with example programs.
PHP – Check if strings are equal ignoring case
To check if two given strings are equal ignoring case in PHP, use strcasecmp()
function and pass the two strings as arguments.
If both the strings are equal in value ignoring the case, then strcasecmp()
function returns an integer value of 0
, else it returns a non-zero value.
The boolean expression to check if two strings $x
and $y
are equal ignoring the case is
strcasecmp($x, $y) == 0
This expression can be used in a conditional statement like if, if-else, etc.
Examples
1. Positive Scenario (Equal strings ignoring case)
In the following example, we take string values: $x
and $y
, and check if they are equal.
We have taken the string values such that they are of equal value ignoring the case. Therefore, based on the program and given values, if-block must run.
PHP Program
<?php
$x = "apple";
$y = "APPLE";
if ( strcasecmp($x, $y) == 0 ) {
echo "strings are equal ignoring case";
} else {
echo "strings are not equal ignoring case";
}
?>
Output
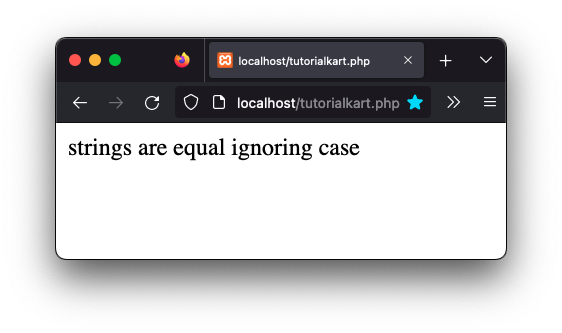
2. Negative Scenario (Not equal strings)
In the following example, we take different string values in $x and $y, and check if they are equal ignoring the case.
Since the string values are not equal in value even if the case of the character is ignored, else-block must run.
PHP Program
<?php
$x = "apple";
$y = "Banana";
if ( strcasecmp($x, $y) == 0 ) {
echo "strings are equal ignoring case";
} else {
echo "strings are not equal ignoring case";
}
?>
Output
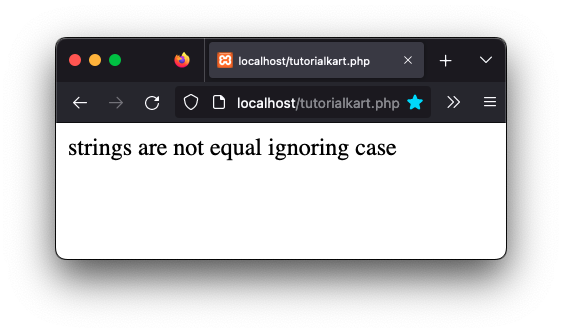
Conclusion
In this PHP Tutorial, we learned how to check if two given strings are equal or not by ignoring the case, using strcasecmp() function, with the help of example programs.