In this PHP tutorial, you shall learn how to compare given two strings using strcmp() function, with example programs.
PHP – Compare Strings
To compare strings in PHP, you can use strcmp() function. strcmp() can find if a string is smaller, equal to, or larger than the other string.
The syntax of strcmp() function to compare $string1 and $string2 is
result = strcmp($string1, $string2)
strcmp() compares strings lexicographically.
- If $string1 precedes $string2 when arranged lexicographically in ascending order, $string1 is said to be smaller than $string2. In this case, strcmp($string1, $string2) returns negative value.
- If $string1 comes after $string2 when arranged lexicographically in ascending order, $string1 is said to be larger than $string2. In this case, strcmp($string1, $string2) returns positive value.
- If $string1 equals $string2, then strcmp($string1, $string2) returns 0.
Examples
1. Compare strings, given string1 is less than string2
In this example, we will take two strings: “apple”, “banana”. First string comes prior to second string when arranged in ascending order lexicographically. So, when we pass these two strings as arguments to strcmp(), it should return a negative value.
PHP Program
<?php
$string_1 = "apple";
$string_2 = "banana";
$result = strcmp($string_1, $string_2);
echo $result;
?>
Output
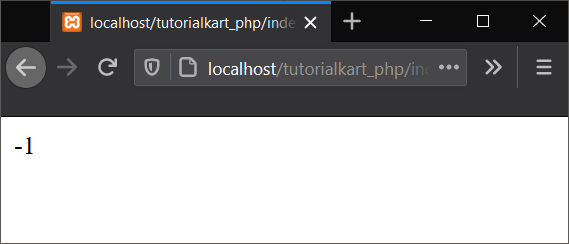
2. Compare strings, given string1 is equal to string2
In this example, we will take two strings: “apple”, “apple”. Both the strings are equal. So, when we pass these two strings as arguments to strcmp(), it should return a value of zero.
PHP Program
<?php
$string_1 = "apple";
$string_2 = "apple";
$result = strcmp($string_1, $string_2);
echo $result;
?>
Output
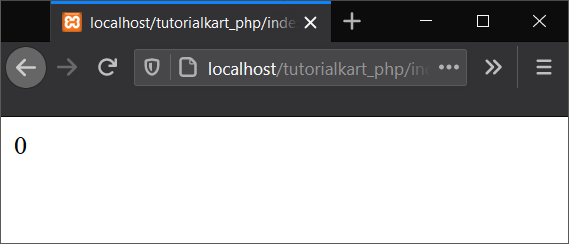
3. Compare strings, given string1 is greater than string2
In this example, we will take two strings: “orange”, “apple”. First string comes after the second string when arranged in ascending order lexicographically. So, when we pass these two strings as arguments to strcmp(), it should return a positive value.
PHP Program
<?php
$string_1 = "orange";
$string_2 = "apple";
$result = strcmp($string_1, $string_2);
echo $result;
?>
Output
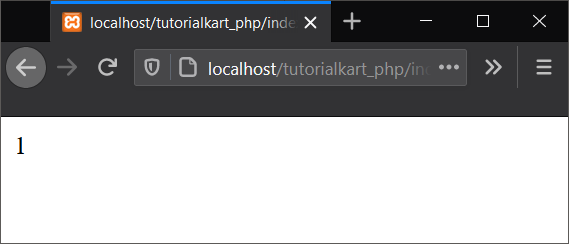
Conclusion
In this PHP Tutorial, we learned how to compare two strings in PHP using strcmp() built-in function.